I picked up a
cheap bluetooth serial (rs232) module from Amazon. As it didn't cost too much, I thought I'd take a chance. The module arrived fine, though (unsurprisingly) without any documentation. Initially I'd tried following the documentation for
configuring a HC-05 module, but after I while I realised it was actually a HC-06 module. The HC-06 is very similar to the HC-05, but (crucially) initially runs at a different baud rate (9600bps instead of 38400bps). Luckily I found some good instructions for
configuring the HC-06 serial module elsewhere. I'm going to recap what I did to get the module working with my (OS X) laptop and a
USB serial adapter. Initially I just connected the pins from the bluetooth module to the USB serial adapter directly. Although this meant that the power/ground pins lined up it also meant that the rx/tx (receive/transmit) pins also lined up - which we don't actually want. We actually want the rx pin on the serial adapter to go to the tx pin on the bluetooth adapter (and vice-versa). This is because it's not simply passing through the signals - instead we're transmitting from the serial and receiving on the bluetooth adapter. This also means that we have to make sure the baud rates of both adapters match. You can see the pinouts of the bluetooth (top) and serial (bottom) adapters here:
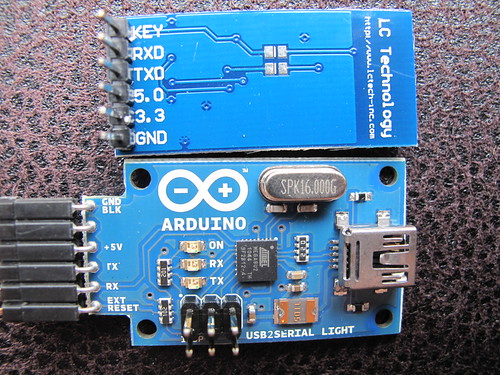
I used a breadboard and some jumper wires so I could have rx and tx swapped over:

I also connected the 5v/power of the serial adapter to the 5v/power pin of the bluetooth adapter and did the same for ground. The other pins I left unattached (labelled "key" and "3v" on the bluetooth adapter). At this point I was then able to use the
Serial Monitor from within the
Arduino IDE to send
AT commands to the bluetooth module. The key thing for this adapter was to ensure that the baud rate was set to 9600bps and the serial monitor was configured
not to send line endings (cr/lf). This particular adapter expects to receive the full commands in one go, which works well with the serial monitor in the Arduino IDE, as it lets us type text then send it when we're ready. Here are some screen shots of using the serial adapter to configure the bluetooth adapter with AT commands - via the Arduino IDEs serial monitor (Note how the baud rate is set to 9600 and "No line ending" is selected). Firstly here I just send the command "AT":
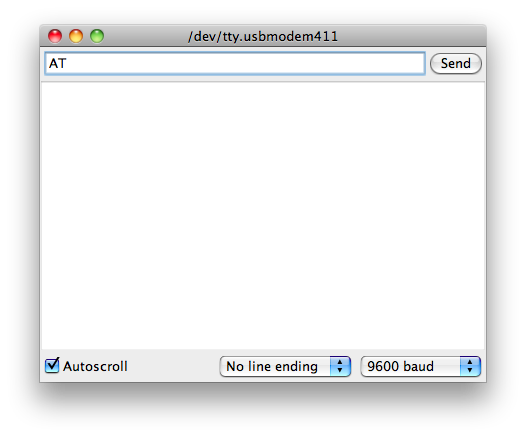
Then the adapter responds back with "OK":
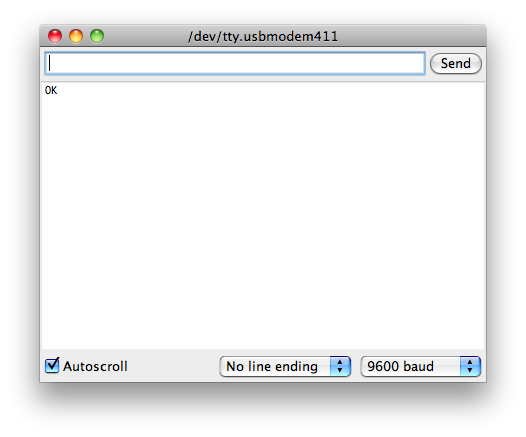
This command does nothing, but it's handy for making sure that things are at least connected properly. Then here are some shots of changing the bluetooth adapter's name to "box-bot" by sending the command "AT+NAMEbot-box":
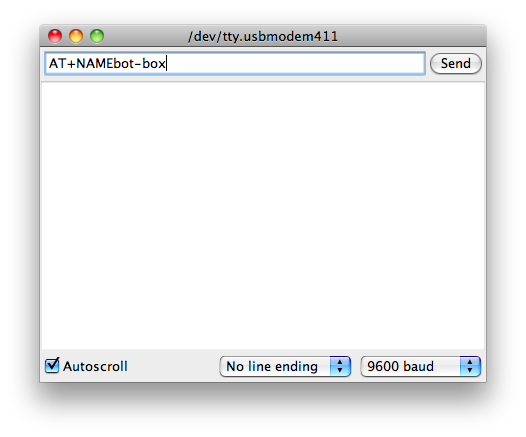
Which yields a reply of "OKsetname":
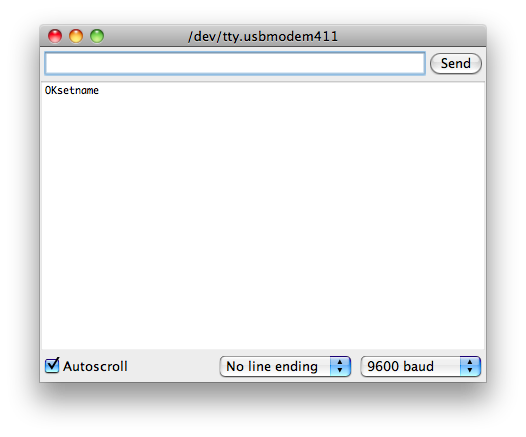
At this point the adapter's name has been changed to "box-bot". So now we can attempt to communicate with it over bluetooth (rather than via the serial port).
So the next job is to "pair" the module with my OS X laptop using the "Bluetooth Setup Assistant" app. We start up the Bluetooth Setup Assistant and we can see the "box-bot" module listed:
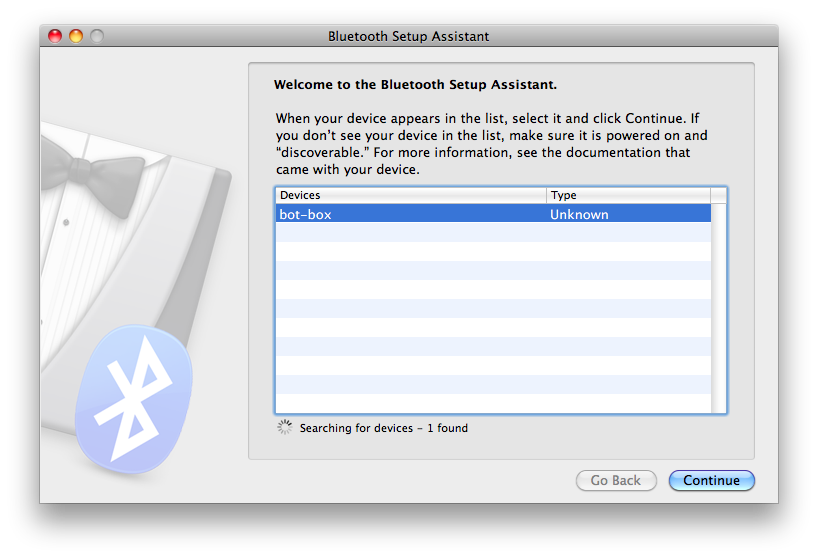
Select the adapter from the list and click "Continue". Then we get to choose how to pair the adapter. In our case we want to enter a passcode:
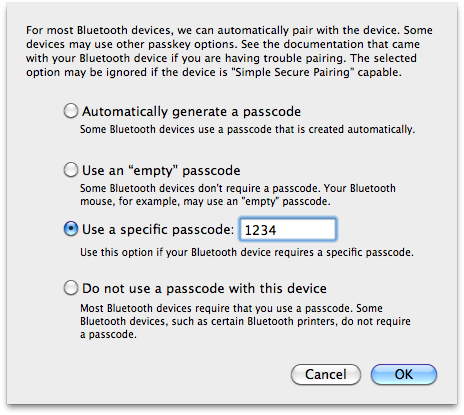
The default passcode is normally "0000", but can actually be changed using "AT+PIN1234" (which would set the code to 1234).
Hopefully you should then see the following, indicating that pairing has worked:
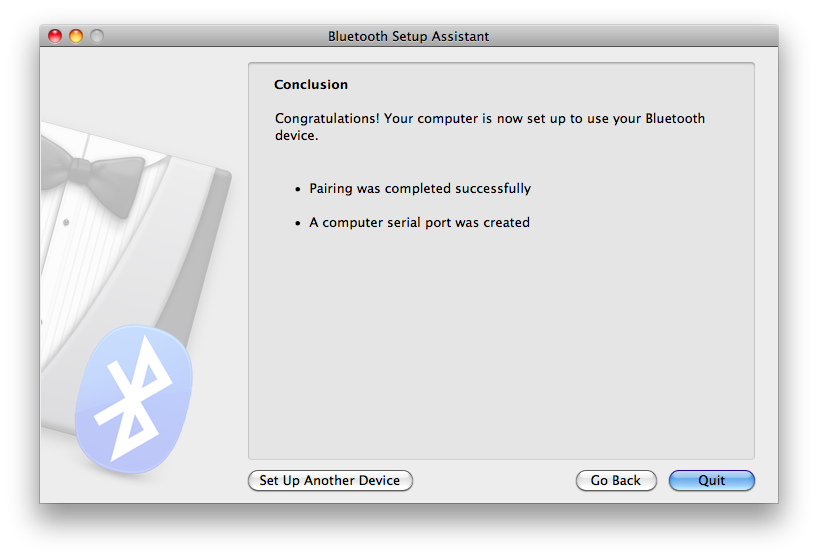
This should hopefully then mean you have a new serial port file descriptor created in /dev. In my case this is named /dev/tty.bot-box-DevB and this is what you'd use in any code to connect to the serial port.
So in Python, using the pyserial module you'd do something like this:
import serial
port = serial.Serial('/dev/tty.bot-box-DevB',
baudrate=9600, timeout=0)
Though you could use a the comports function to give you a list of all available serial ports and pick from the list yourself.
I did find that to get the serial port to properly work you first had to scan for available Bluetooth devices (e.g. by running just the first step of the Bluetooth Setup Assistant). Otherwise connecting to the /dev/tty.bot-box-DevB serial port would report an error. I guess it's a bit like plugging in a cable. Once the connection is established though it's essentially the same as a physical serial port (from a code point of view), without the need for a physical wire. This makes it great for things like the Arduino, where the serial port is a pretty standard way to communicate with a main computer.
The only odd thing was it seemed the connection tended to eat newline (\n) characters. Not 100% why this would be the case, but it's something to watch out for when using this adapter.